7.11. Blazed gratings
In this example we show that blazed grating generates order 1, only when the angle of the grating is the correct one (maximum phase shift = 2\(\pi\)). On the other hand, for other grating angle, several diffraction orders are generated.
[3]:
from diffractio import np, plt
from diffractio import um, mm, degrees
from diffractio.scalar_masks_X import Scalar_mask_X
from diffractio.scalar_masks_XY import Scalar_mask_XY
from diffractio.scalar_sources_XY import Scalar_source_XY
from diffractio.scalar_fields_XZ import Scalar_field_XZ
7.11.1. Grating in x mode
Grating with correct phase
[4]:
wavelength = 1 * um
focal = 5 * mm
x = np.linspace(-3500,3500,2048*8)
z = np.linspace(focal - 2 * mm, focal + 2 * mm, 128)
[5]:
t_lens_x = Scalar_mask_X(x, wavelength)
t_lens_x.lens(x0=0, focal=focal, radius=0)
[6]:
t_blazed_x = Scalar_mask_X(x, wavelength)
t_blazed_x.blazed_grating(x0=125, period=10, phase_max=2 * np.pi)
[7]:
u1 = t_lens_x * t_blazed_x
u2 = u1.RS(z=focal)
u2.draw()
plt.xlim(-2000, 2000)
Good result: factor 2.04
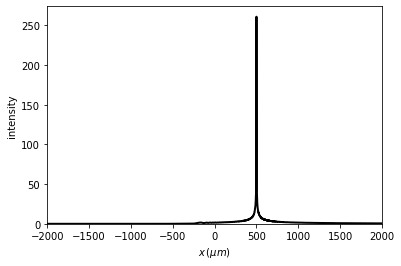
Grating with wrong phase
[8]:
t_blazed2_x = Scalar_mask_X(x, wavelength)
t_blazed2_x.blazed_grating(x0=125, period=10, phase_max=1.5 * np.pi)
[19]:
u1_x = t_lens_x * t_blazed2_x
u2_x = u1_x.RS(z=focal)
u2_x.draw()
plt.xlim(-2000, 2000)
Good result: factor 2.04
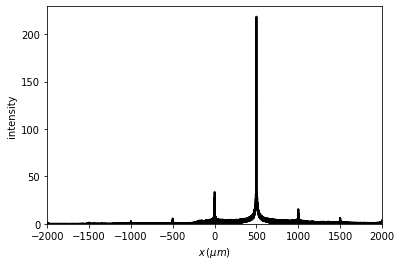
[10]:
txz=Scalar_field_XZ(x=x, z=z, wavelength=wavelength)
txz.incident_field(u1_x)
txz.RS()
txz.draw(logarithm=5)
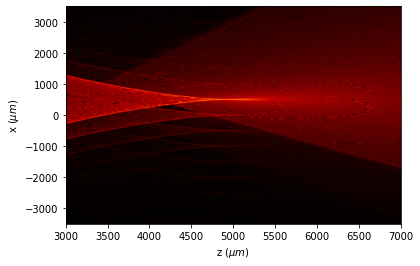
7.11.2. Grating in XY mode
Grating with correct phase
[11]:
num_pixels = 512
length = 500 * um
x0 = np.linspace(-length / 2, length / 2, num_pixels)
y0 = np.linspace(-length / 2, length / 2, num_pixels)
wavelength = 0.6238 * um
focal = 5 * mm
[12]:
u0_xy = Scalar_source_XY(x=x0, y=y0, wavelength=wavelength)
u0_xy.plane_wave(A=1)
u0_xy.pupil()
[13]:
t_lens_xy = Scalar_mask_XY(x=x0, y=y0, wavelength=wavelength)
t_lens_xy.lens(r0=(0, 0), focal=focal)
[14]:
t_blazed_xy = Scalar_mask_XY(x=x0, y=y0, wavelength=wavelength)
t_blazed_xy.blazed_grating(period=20 * um,
phase_max=2 * np.pi,
x0=0 * um,
angle=0 * degrees)
[15]:
u1_xy = u0_xy * t_lens_xy * t_blazed_xy
u2_xy = u1_xy.RS(z=focal, new_field=True, amplification=(3, 1))
u2_xy.draw(logarithm=1e1)
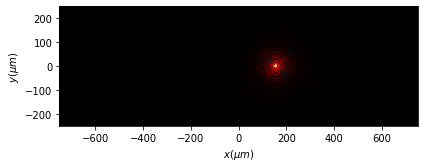
Grating with wrong phase
[16]:
t_blazed2_xy = Scalar_mask_XY(x=x0, y=y0, wavelength=wavelength)
t_blazed2_xy.blazed_grating(period=20 * um,
phase_max=1.5 * np.pi,
x0=0 * um,
angle=0 * degrees)
[17]:
u1_xy = u0_xy * t_lens_xy * t_blazed2_xy
u2_xy = u1_xy.RS(z=focal, new_field=True, amplification=(3, 1))
u2_xy.draw(logarithm=1e1)
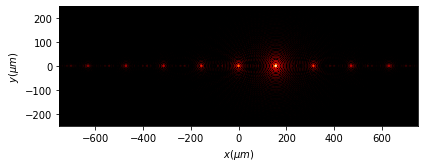